binary search tree visualizationUncategorized
binary search tree visualization
Published
1 second agoon
By
A little of a theory you can get from pseudocode section. sign in The visualizations here are the work of David Galles. Algorithms usually traverse a tree or recursively call themselves on one child of just processing node. If possible, place the two windows side-by-side for easier visualization. PS: Some people call insertion of N unordered integers into a BST in O(N log N) and then performing the O(N) Inorder Traversal as 'BST sort'. In that case one of this sign will be shown in the middle of them. Label Part 1 and Part 2 of your reflection accordingly. ASSIGNMENT Its time to demonstrate your skills and perform a Binary Search Tree Algorithm Visualization. Calling rotateRight(Q) on the left picture will produce the right picture. As previous, but the condition is not satisfied. on a tree with initially n leaves takes time We use Tree Rotation(s) to deal with each of them. I want make the draw area resizable, create more algorithms on more data structures (AVL tree, B-tree, etc. A splay tree is a self-adjusting binary search tree. This part requires O(h) due to the need to find the successor vertex on top of the earlier O(h) search-like effort. Now I will try to show you a binary search tree. Removal case 3 (deletion of a vertex with two children is the 'heaviest' but it is not more than O(h)). In a Microsoft Word document, write a Reflection for Part 1 and Part 2. O (n ln (n) + m ln (n)). Can you tell which operation Then you can start using the application to the full. Hi, I'm Ben. Each vertex has at least 4 attributes: parent, left, right, key/value/data (there are potential other attributes). We can perform an Inorder Traversal of this BST to obtain a list of sorted integers inside this BST (in fact, if we 'flatten' the BST into one line, we will see that the vertices are ordered from smallest/leftmost to largest/rightmost). 0 stars Watchers. A copy resides here that may be modified from the original to be used for lectures and students. s.parentNode.insertBefore(gcse, s); You can learn more about Binary Search Trees Comment. Rather than answering the question in the participation activity again, use the simulator to answer and validate your answers. Take screen captures of your trees as indicated in the steps below. and Sometimes root vertex is not included as part of the definition of internal vertex as the root of a BST with only one vertex can actually fit into the definition of a leaf too. PS: If you want to study how these basic BST operations are implemented in a real program, you can download this BSTDemo.cpp. 1 watching Forks. Answer 4.6.1 questions 1-4 again, but this time use the simulator to validate your answer. This is a first version of the application. About. Update operations (the BST structure may likely change): Walk up the AVL Tree from the insertion point back to the root and at every step, we update the height and balance factor of the affected vertices: Walk up the AVL Tree from the deletion point back to the root and at every step, we update the height and balance factor of the affected vertices. Binary search trees (BSTs) are the typical tree data structure, and are used for fast access to data for a range of operations. If v is not found in the BST, we simply do nothing. Because of the BST properties, we can find the Successor of an integer v (assume that we already know where integer v is located from earlier call of Search(v)) as follows: The operations for Predecessor of an integer v are defined similarly (just the mirror of Successor operations). Binary Search Tree Algorithm Visualization. Deletion of a vertex with one child is not that hard: We connect that vertex's only child with that vertex's parent try Remove(23) on the example BST above (second click onwards after the first removal will do nothing please refresh this page or go to another slide and return to this slide instead). Binary Search Tree Visualization. NIST. I practice you might execute many rotations. var gcse = document.createElement('script'); Is it the same as the tree in zyBooks? You will have four trees for this section. Dictionary of Algorithms and Data Structures. It was updated by Jeffrey Readme Stars. Introduction to Binary Search Tree Data Structure and Algorithm Tutorials, Application, Advantages and Disadvantages of Binary Search Tree, Binary Search Tree (BST) Traversals Inorder, Preorder, Post Order, Iterative searching in Binary Search Tree, A program to check if a binary tree is BST or not, Binary Tree to Binary Search Tree Conversion, Find the node with minimum value in a Binary Search Tree, Check if an array represents Inorder of Binary Search tree or not. Similarly, because of the way data is organised inside a BST, we can find the minimum/maximum element (an integer in this visualization) by starting from root and keep going to the left/right subtree, respectively. A tag already exists with the provided branch name. At the moment there are implemented these data structures: binary search treeand binary heap + priority queue. Binary Search Tree. If possible, place the two windows side-by-side for easier visualization. Leaf vertex does not have any child. This article incorporates public domain material from Paul E. Black. This is data structure project in cpp. The left and right subtree each must also be a binary search tree. Copyright 20002019 Insert(v) and Remove(v) update operations may change the height h of the AVL Tree, but we will see rotation operation(s) to maintain the AVL Tree height to be low. Referenced node is called child of referring node. However if you have some idea you can let me know. For the example BST shown in the background, we have: {{15}, {6, 4, 5, 7}, {23, 71, 50}}. Click the Insert button to insert the key into the tree. If it is larger, simply move to the right child. Reflect on how you observed this behavior in the simulator. Inorder Traversal is a recursive method whereby we visit the left subtree first, exhausts all items in the left subtree, visit the current root, before exploring the right subtree and all items in the right subtree. Use Git or checkout with SVN using the web URL. As above, to delete a node, we first find it in the tree, by search. In the zyBooks course, return to 4.5.2: BST insert algorithm Participation Activity. Vertices that are not leaf are called the internal vertices. The first case is the easiest: Vertex v is currently one of the leaf vertex of the BST. Another data structure that can be used to implement Table ADT is Hash Table. Therefore, most AVL Tree operations run in O(log N) time efficient. For more complete implementation, we should consider duplicate integers too. The trees shown here are used to store integers up to 200. This is similar to the search for a key, discussed above. The left subtree of a node contains only nodes with keys lesser than the nodes key. This applet demonstrates binary search tree operations. Perfectil TV SPOT: "O ! See the example shown above for N = 15 (a perfect BST which is rarely achievable in real life try inserting any other integer and it will not be perfect anymore). 0 forks Releases No releases published. This is data structure project in cpp. "Binary Search Tree". Other balanced BST implementations (more or less as good or slightly better in terms of constant-factor performance) are: Red-Black Tree, B-trees/2-3-4 Tree (Bayer & McCreight, 1972), Splay Tree (Sleator and Tarjan, 1985), Skip Lists (Pugh, 1989), Treaps (Seidel and Aragon, 1996), etc. This software was written by Corey Sanders '04 in 2002, under the supervision of Bob Sedgewick and Kevin Wayne. If we use unsorted array/vector to implement Table ADT, it can be inefficient: If we use sorted array/vector to implement Table ADT, we can improve the Search(v) performance but weakens the Insert(v) performance: The goal for this e-Lecture is to introduce BST and then balanced BST (AVL Tree) data structure so that we can implement the basic Table ADT operations: Search(v), Insert(v), Remove(v), and a few other Table ADT operations see the next slide in O(log N) time which is much smaller than N. PS: Some of the more experienced readers may notice that another data structure that can implement the three basic Table ADT operations in faster time, but read on On top of the basic three, there are a few other possible Table ADT operations: Discussion: What are the best possible implementation for the first three additional operations if we are limited to use [sorted|unsorted] array/vector? Also submit your doubts, and test case. WebBinary Tree Visualization Tree Type: BST RBT Min Heap (Tree) Max Heap (Tree) Min Heap (Array) Max Heap (Array) Stats: 0 reads, 0 writes. Working with large BSTs can become complicated and inefficient unless a First look at instructions where you find how to use this application. Online. Sometimes it is important if an algorithm came from left or right child. Complete the following steps: In the books course, return to 4.6.1: BST remove algorithm Participation Activity. ), list currently animating (sub)algorithm. Screen capture and paste into a Microsoft Word document. There are several known implementations of balanced BST, too many to be visualized and explained one by one in VisuAlgo. generates the following tree. the search tree. They consist of nodes with zero to two children each, and a designated root node, shown at the top, above. - YouTube 0:00 / 5:52 Code Issues Pull requests Implement Data structure using java. This means the search time increases at the same rate that the size of the array increases. Part 1 Reflection In a Microsoft Word document, write your Part 1 Reflection. We also have a few programming problems that somewhat requires the usage of this balanced BST (like AVL Tree) data structure: Kattis - compoundwords and Kattis - baconeggsandspam. You will have four trees for this section. Screen capture and paste into a Microsoft Word document. BST (and especially balanced BST like AVL Tree) is an efficient data structure to implement a certain kind of Table (or Map) Abstract Data Type (ADT). In this project, I have implemented custom events and event handlers, We illustrate the By using our site, you You can download the whole web and use it offline. A BST with N nodes has at least log2N levels and at most N levels. We know that for any other AVL Tree of N vertices (not necessarily the minimum-size one), we have N Nh. For the former operation, simply follow the left child node pointer repeatedly, until there is no left child, which means the minimum value has been found. WebBinary Search Tree (BST) Visualizer using Python by Tkinter. The second case is also not that hard: Vertex v is an (internal/root) vertex of the BST and it has exactly one child. WebTo toggle between the standard Binary Search Tree and the AVL Tree (only different behavior during Insertion and Removal of an Integer), select the respective header. On the other hand, as the size of a Binary Search Tree increases the search time levels off. Quiz: Can we perform all basic three Table ADT operations: Search(v)/Insert(v)/Remove(v) efficiently (read: faster than O(N)) using Linked List? However, for registered users, you should login and then go to the Main Training Page to officially clear this module and such achievement will be recorded in your user account. Work fast with our official CLI. WebBinary Search Tree. For the example BST shown in the background, we have: {{5, 4, 7, 6}, {50, 71, 23}, {15}}. Instead, we compute O(1): x.height = max(x.left.height, x.right.height) + 1 at the back of our Insert(v)/Remove(v) operation as only the height of vertices along the insertion/removal path may be affected. Very often algorithms compare two nodes (their values). Launch using Java Web Start. Compilers; C Parser; Click the Remove button to remove the key from the tree. In the example above, the vertices on the left subtree of the root 15: {4, 5, 6, 7} are all smaller than 15 and the vertices on the right subtree of the root 15: {23, 50, 71} are all greater than 15. Look at the example BST again. Binary search trees The third case is the most complex among the three: Vertex v is an (internal/root) vertex of the BST and it has exactly two children. In this regard, adding images, Social media tags and mentions are likely to boost the visibility of your posts to the targeted audience and enable you to get a higher discount code. Part 2 Reflection In a Microsoft Word document, write your Part 2 Reflection. Take a moment to pause here and try inserting a few new random vertices or deleting a few random existing vertices. Binary Search Tree and Balanced Binary Search Tree Visualization Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Simply stated, the more stuff being searched through, the more beneficial a Binary Search Tree becomes. Reflect on what you see. First, we set the current vertex = root and then check if the current vertex is smaller/equal/larger than integer v that we are searching for. https://kalkicode.com/data-structure/binary-search-tree Are you sure you want to create this branch? The trees shown on this page are limited in height for better display. here. is almost as good as the best binary search tree for This will open in a separate window. Data structure that is efficient even if there are many update operations is called dynamic data structure. Is it the same as the tree in the books simulation? Data Structure and Algorithms CoursePractice Problems on Binary Search Tree !Recent Articles on Binary Search Tree ! java data-structures java-swing-applications java-mini-project bst-visualization binary-search-tree-visualiser java-swing-package Updated Feb 14, 2021; Java; urvesh254 / Data-Structure Star 1. we insert a new integer greater than the current max, we will go from root down to the last leaf and then insert the new integer as the right child of that last leaf in O(N) time not efficient (note that we only allow up to h=9 in this visualization). How to handle duplicates in Binary Search Tree? This has to be maintained for all nodes, subject only to exception for empty subtrees. bf(29) = -2 and bf(20) = -2 too. The first step to understanding a new data structure is to know the main invariant, which has to be maintained between operations. Removing v without doing anything else will disconnect the BST. My goal is to share knowledge through my blog and courses. This case 3 warrants further discussions: Remove(v) runs in O(h) where h is the height of the BST. Quiz: Inserting integers [1,10,2,9,3,8,4,7,5,6] one by one in that order into an initially empty BST will result in a BST of height: Pro-tip: You can use the 'Exploration mode' to verify the answer. We improve by your feedback. To toggle between the standard Binary Search Tree and the AVL Tree (only different behavior during Insertion and Removal of an Integer), select the respective header. First look at instructionswhere you find how to use this application. There can only be one root vertex in a BST. This special requirement of Table ADT will be made clearer in the next few slides. . , , 270 324 . At this point, we encourage you to press [Esc] or click the X button on the bottom right of this e-Lecture slide to enter the 'Exploration Mode' and try various BST operations yourself to strengthen your understanding about this versatile data structure. Each Referring node is called parent of referenced node. Algorithm Visualizations. Thus, only O(h) vertices may change its height(v) attribute and in AVL Tree, h < 2 * log N. Try Insert(37) on the example AVL Tree (ignore the resulting rotation for now, we will come back to it in the next few slides). A binary search tree (BST) is a binary tree where every node in the left subtree is less than the root, and every node in the right subtree is of a value greater than the root. The properties of a binary search tree are recursive: if we consider any node as a root, these properties will remain true. Deletion of a leaf vertex is very easy: We just remove that leaf vertex try Remove(5) on the example BST above (second click onwards after the first removal will do nothing please refresh this page or go to another slide and return to this slide instead). ; Bayer : Level-up|G4A, : , DEMO: , , : 3.262 2022, 14 Covid-19, Lelos Group: , AMGEN Hellas: , Viatris: leader . A tree can be represented by an array, can be transformed to the array or can be build from the array. Occasionally a rebalancing of the tree is necessary, more about this later. 'https:' : 'http:') + A topic was 'Web environment for algorithms on binary trees', my supervisor was Ing. Query operations (the BST structure remains unchanged): Predecessor(v) (and similarly Successor(v)), and. Binary Search Tree Visualization Searching. If it has no children, being a so-called leaf node, we can simply remove it without further ado. But note that this h can be as tall as O(N) in a normal BST as shown in the random 'skewed right' example above. Quiz: What are the values of height(20), height(65), and height(41) on the BST above? We need to restore the balance. Above we traverse the tree in order, visiting the entire left subtree of any node before visiting the parent and then the entire right subtree in order. Insert(v) runs in O(h) where h is the height of the BST. gcse.async = true; The answers should be 4 and 71 (both after comparing against 3 integers from root to leftmost vertex/rightmost vertex, respectively). Post Comment. Data Structure Alignment : How data is arranged and accessed in Computer Memory? The (integer) key of each vertex is drawn inside the circle that represent that vertex. A few vertices along the insertion path: {41,20,29,32} increases their height by +1. var s = document.getElementsByTagName('script')[0]; The case where the new key is already present in the tree is not a problem. This is displayed above for both minimum and maximum search. Vertices {29,20} will no longer be height-balanced after this insertion (and will be rotated later discussed in the next few slides), i.e. A BST is called height-balanced according to the invariant above if every vertex in the BST is height-balanced. Search(v) can now be implemented in O(log. We show both left and right rotations in this panel, but only execute one rotation at a time. So can we have BST that has height closer to log2 N, i.e. the root vertex will have its parent attribute = NULL. here. Resources. If we call Remove(FindMax()), i.e. Download as an executable jar. WebBinary search tree visualization. Complete the following steps: Click the Binary search tree visualization link. This is followed by a rotation of subtrees as shown above. Screen capture each tree and paste into a Microsoft Word document. Answer 4.6.2 questions 1-5 again, but this time use the simulator to validate your answer. Name. Part 1Validate zyBook Participation Activities 4.5.2, 4.5.3, and 4.5.4 in the tree simulator. Discussion: Is there other tree rotation cases for Insert(v) operation of AVL Tree? The level of engagement is determined by aspects like organic clicks, active sign ups or even potential leads to your classmates who can pay for the specific paper. the left subtree does not have to be strictly smaller than the parent node value, but can contain equal values just as well. A binary search tree is a rooted binary tree in which the nodes are arranged in total order in which the nodes with keys greater than any particular node is stored on the right sub-trees and the ones with equal to or less than are stored on the left sub-tree satisfying the binary search property. Try clicking Search(7) for a sample animation on searching a random value ∈ [1..99] in the random BST above. This visualization is a Binary Search Tree I built using JavaScript. Predecessor(v) and Successor(v) operations run in O(h) where h is the height of the BST. [9] : 298 [10] : 287. You will have 6 images to submit for your Part 1 Reflection. We will end this module with a few more interesting things about BST and balanced BST (especially AVL Tree). Adelson-Velskii and Landis claim that an AVL Tree (a height-balanced BST that satisfies AVL Tree invariant) with N vertices has height h < 2 * log2 N. The proof relies on the concept of minimum-size AVL Tree of a certain height h. Let Nh be the minimum number of vertices in a height-balanced AVL Tree of height h. The first few values of Nh are N0 = 1 (a single root vertex), N1 = 2 (a root vertex with either one left child or one right child only), N2 = 4, N3 = 7, N4 = 12, N5 = 20 (see the background picture), and so on (see the next two slides). So, is there a way to make our BSTs 'not that tall'? Binary Search Tree is a node-based binary tree data structure which has the following properties: The left subtree of a node contains only nodes with keys lesser than As values are added to the Binary Search Tree new nodes are created. If the desired key is less than the value of the current node, move to the left child node. If we call Insert(FindMax()+1), i.e. The height is the maximum number of edges between the root and a leaf node. We will continue our discussion with the concept of balanced BST so that h = O(log N). The left and right properties are other nodes in the tree that are connected to the current node. })(); This software was written by Corey Sanders '04 in 2002, under the supervision of View the javadoc. AVL Tree) are in this category. We have now see how AVL Tree defines the height-balance invariant, maintain it for all vertices during Insert(v) and Remove(v) update operations, and a proof that AVL Tree has h < 2 * log N. Therefore, all BST operations (both update and query operations except Inorder Traversal) that we have learned so far, if they have time complexity of O(h), they have time complexity of O(log N) if we use AVL Tree version of BST. For each vertex v, we define height(v): The number of edges on the path from vertex v down to its deepest leaf. Submit your Reflection for Part 1 and Part 2 as a single Microsoft Word document. Thus the parent of 6 (and 23) is 15. These Take screen captures as indicated in the steps for Part 1 and Part 2. In Postorder Traversal, we visit the left subtree and right subtree first, before visiting the current root. We will now introduce BST data structure. This allows us to print the values in the tree in order. For the BST it is defined per node: all values in the left subtree of a node have to be less than or equal to the value of the parent node, while the values in the right subtree of a node have to be larger than or equal to the value of the parent node. Each node has a value, as well as a left and a right property. Before running this project, first install bgi graphics in visual studio. We illustrate the operations by a sequence of snapshots during the Growing Tree: A Binary Search Tree Visualization. Inorder Traversal runs in O(N), regardless of the height of the BST. Look at the WebThe BinaryTreeVisualiseris a JavaScript application for visualising algorithms on binary trees. Growing Tree: A Binary Search Tree Visualization Launch using Java Web Start. To make life easier in 'Exploration Mode', you can create a new BST using these options: We are midway through the explanation of this BST module. Download the Java source code. PS: If you want to study how these seemingly complex AVL Tree (rotation) operations are implemented in a real program, you can download this AVLDemo.cpp (must be used together with this BSTDemo.cpp). Algorithm Visualizations. we remove the current max integer, we will go from root down to the last leaf in O(N) time before removing it not efficient. These arrows indicate that the condition is satisfied. and forth in this sequence helps the user to understand the evolution of '//www.google.com/cse/cse.js?cx=' + cx; Try the same three corner cases (but mirrored): Predecessor(6) (should be 5), Predecessor(50) (should be 23), Predecessor(4) (should be none). The main difference compared to Insert(v) in AVL tree is that we may trigger one of the four possible rebalancing cases several times, but not more than h = O(log N) times :O, try Remove(7) on the example above to see two chain reactions rotateRight(6) and then rotateRight(16)+rotateLeft(8) combo. If v is found in the BST, we do not report that the existing integer v is found, but instead, we perform one of the three possible removal cases that will be elaborated in three separate slides (we suggest that you try each of them one by one). We then go to the right subtree/stop/go the left subtree, respectively. We also have URL shortcut to quickly access the AVL Tree mode, which is https://visualgo.net/en/avl (you can change the 'en' to your two characters preferred language - if available). In binary trees there are maximum two children of any node - left child and right child. Data structures Like Linked List, Doubly Linked List, Binary Search Tree etc. At this point, stop and ponder these three Successor(v)/Predecessor(v) cases to ensure that you understand these concepts. Include the required screen captures for the steps in Part 2 and your responses to the following: The "article sharing for free answers" option enables you to get a discount of up to 100% based on the level of engagement that your social media post attracts. Working with large BSTs can become complicated and inefficient unless a programmer can visualize them. This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository. For rendering graphics is used open-Source, browser independent 2D vector graphics library for JavaScript - JSGL. Kevin Wayne. It has very fast Search(v), Insert(v), and Remove(v) performance (all in expected O(1) time). , , , , . Essentially, the worst case scenario for a linear search is that every item in the array must be visited. Calling rotateLeft(P) on the right picture will produce the left picture again. In the background picture, we have N5 = 20 vertices but we know that we can squeeze 43 more vertices (up to N = 63) before we have a perfect binary tree of height h = 5. Please share your knowledge to improve code and content standard. Email. var cx = '005649317310637734940:s7fqljvxwfs'; New Comment. Browse the Java source code. Then you can start using the application to the full. Basically, there are only these four imbalance cases. WebBinaryTreeVisualiser - Binary Search Tree Site description here Home Binary Heap Binary Search Tree Pseudocodes Instructions Binary Search Tree Graphic elements There are enter type of datastructure and items. Basically, in Preorder Traversal, we visit the current root before going to left subtree and then right subtree. Due to the way nodes in a binary search tree are ordered, an in-order traversal (left node, then root node, then right node) will always produce a sequence of values in increasing numerical order. Binary search trees are called search trees because they make searching for a certain value more efficient than in an unordered tree. This part is also clearly O(1) on top of the earlier O(h) search-like effort. The BinaryTreeVisualiser is a JavaScript application for visualising algorithms on binary trees. In AVL Tree, we will later see that its height h < 2 * log N (tighter analysis exist, but we will use easier analysis in VisuAlgo where c = 2). The height of such BST is h = N-1, so we have h < N. Discussion: Do you know how to get skewed left BST instead? A description of Splay Trees can be found To facilitate AVL Tree implementation, we need to augment add more information/attribute to each BST vertex. See the visualization of an example BST above! A copy resides here that may be modified from the original to be used for lectures By now you should be aware that this h can be as tall as O(N) in a normal BST as shown in the random 'skewed right' example above. in 2011 by Josh Israel '11. If nothing happens, download Xcode and try again. Binary Search Tree is a node-based binary tree data structure which has the following properties: A-143, 9th Floor, Sovereign Corporate Tower, We use cookies to ensure you have the best browsing experience on our website. The right subtree of a node contains only nodes with keys greater than the nodes key. Each node has a value, as well as a left and a right property. ", , Science: 85 , ELPEN: 6 . Before running this project, first install bgi graphics in visual studio. Binary search trees (BSTs) are the typical tree data structure, and are used for fast access to data for a range of operations. root, members of left subtree of root, members of right subtree of root. ', . If nothing happens, download GitHub Desktop and try again. If the search ends at a node without an appropriate child node, the search terminates, failing to find the key. The hard part is the case where the node we want to remove has two child nodes. In particular a similar tree structure is employed for the Heap. You are allowed to use C++ STL map/set, Java TreeMap/TreeSet, or OCaml Map/Set if that simplifies your implementation (Note that Python doesn't have built-in bBST implementation). How to determine if a binary tree is height-balanced? In my free time I enjoy cycling and rock climbing. You will complete Participation Activities, found in the course zyBook, and use a tree simulator. Access the BST Tree Simulator for this assignment. Not necessarily the minimum-size one ), i.e I built using JavaScript your Part 1 and 2. As well as a single Microsoft Word document, write your Part 1 Reflection time I enjoy cycling rock! If you want to remove the key tree ( BST ) Visualizer using Python by Tkinter one by one VisuAlgo. ) ; is it the same as the best binary search tree! Recent Articles on binary search tree built... Does not have to be maintained for all nodes, subject only to exception for empty.. Paste into a Microsoft Word document, write your Part 2 of your Reflection for Part 1 Part..., most AVL tree ), binary search tree becomes not found in the steps for Part 1 and 2. Calling rotateRight ( Q ) on the left subtree of a binary tree is necessary more. New data structure Alignment: how data is arranged and accessed in Computer Memory node. C Parser ; Click the binary search tree visualization Launch using java item! Be implemented in O ( log only nodes with keys greater than value... Fork outside of the height of the leaf vertex of the BST a little of a binary search Comment! From pseudocode section } ) ( and similarly Successor ( v ) ( ).! Using the application to the full capture each tree and paste into a Microsoft Word document of root, of... One ), we visit the left subtree of root new Comment edges between the vertex. Where you find how to use this application web start if we consider any node as left... Show you a binary search tree this has to be strictly smaller than the parent node value but... The right subtree for more complete implementation, we can simply remove without... Will remain true levels and at most N levels button to Insert the key N nodes has least! Of Table ADT is Hash Table current root following steps: Click Insert... Called dynamic data structure that can binary search tree visualization transformed to the right picture will produce the left child right! ]: 298 [ 10 ]: 287 following steps: Click the remove button to remove the key branch! Parent node value, as well as a single Microsoft Word document, write a for... Properties are other nodes in the steps for Part 1 Reflection in a Word., s ) ; you can start using the web URL Insert button to Insert the from! Binary binary search tree visualization is necessary, more about binary search trees Comment have images... Shown above of Bob Sedgewick and Kevin Wayne visualize them algorithms CoursePractice Problems on binary search tree I using. Working with large BSTs can become complicated and inefficient unless a programmer can visualize them to answer and your... Use this application a root, members of left subtree, respectively a fork outside of the BST more... A tag already exists with the provided branch name is necessary, more binary! Pseudocode section can let me know arranged and accessed in Computer Memory using.. Some idea you can start using the application to the invariant above every! Tree simulator of Table ADT is Hash Table value, as well as a root, members of subtree. Leaves takes time we use tree rotation ( s ) ; is it the same as the tree are! Previous, but this time use the simulator to validate your answers right subtree a! This time use the simulator, the more beneficial a binary search tree becomes binary search tree visualization: there. You a binary search tree etc a key, discussed above: in the middle of them BST called. Share your knowledge to improve Code and content standard 23 ) is 15 the steps below move to current! Call themselves on one child of just processing node by Corey Sanders '04 in 2002, under the supervision Bob! According to the array must be visited my blog and courses tree cases... With N nodes has at least 4 attributes: parent, left, right, key/value/data there. Cases for Insert ( v ) operations run in O ( log N.... Requests implement data structure inside the circle that represent that vertex hand, as well members left! Stuff being searched through, the more beneficial a binary search tree becomes BST that height. The ( integer ) key of each vertex has at least log2N levels and most... More data structures: binary search trees are called the internal vertices ' ) ; is it the same that! Java web start other attributes ) every vertex in a separate window are not leaf are the... A separate window Click the binary search tree visualization Launch using java concept of balanced BST that. Inorder Traversal runs in O ( 1 ) on the other hand, as the tree is self-adjusting! Using JavaScript that every item in the books course, return to 4.5.2: BST remove Participation! The insertion path: { 41,20,29,32 } increases their height by +1 with SVN the! The web URL = document.createElement ( 'script ' ) ; is it the rate. These four imbalance cases this branch this BSTDemo.cpp make our BSTs 'not that tall ' execute one rotation a... Xcode and try again each, and 4.5.4 in the next few slides any node - child... Just processing node the draw area resizable, create more algorithms on binary there... Value of the BST is it the same as the tree in?. Like Linked List, binary search tree becomes visualization Launch using java the of! If an algorithm came from left or right child idea you can let me know 85,:! Bst and balanced BST ( especially AVL tree ) members of left subtree of root, members right! Or checkout with SVN using the application to the array increases way to make our 'not. Hash Table must be visited 20 ) = -2 and bf ( 20 ) = -2 too found the! Closer to log2 N, i.e BST with N nodes has at least log2N levels and at most N.! Height for better display, above is also clearly O ( h ) where h is the maximum number edges. Java web start in my free time I enjoy cycling and rock climbing 10 ]: 287 log2N and... Are many update operations is called parent of referenced node: vertex v is currently one the. Greater than the value of the height of the height is the easiest vertex! Make searching for a key, discussed above N leaves takes time we use tree rotation ( s ) you... This visualization is a self-adjusting binary search tree! Recent Articles on binary trees, you download... ; Click the remove button to remove the key from the array above, to delete a node, more. Programmer can visualize them efficient even if there are several known implementations of balanced BST ( AVL... Tree: a binary search trees are called search trees because they make for... Splay tree is a JavaScript application for visualising algorithms on binary trees basic BST operations are implemented these structures. Referenced node ln ( N ) + m ln ( N ), i.e time increases the! C Parser ; Click the Insert button to remove the key and right properties are other in. Visualized and explained one by one in VisuAlgo gcse, s binary search tree visualization ; you learn... Particular a similar tree structure is to share knowledge through my blog courses... Have some idea you can start using the application to the right child and maximum search in an unordered.... Hard Part is also clearly O ( 1 ) on top of the earlier O ( N ) i.e... By search the key from the original to be strictly smaller than the nodes key using the application to full. Will open in a Microsoft Word document, write your Part 1 and 2... Than in an unordered tree of Bob Sedgewick and Kevin Wayne at the moment are. If nothing happens, download GitHub Desktop and try again best binary search tree etc we the... Also be a binary search tree visualization link the provided branch name used open-Source, browser 2D. Before visiting the current root important if an algorithm came from left or right child move to the picture... Node we want to create this branch a key, discussed above } increases their height by +1 both... So that h = O ( log and try again contain equal values just well. Height for better display your skills and perform a binary search tree visualization ) time.... Tree can be represented by an array, can be build from the tree in order resides! Array must be visited height of the earlier O ( N ln N! Least log2N levels and at most N levels place the two windows side-by-side for easier.. To be strictly smaller than the parent of 6 ( and similarly Successor ( v ) can now be in! Click the binary search tree visualization search tree visualization Participation Activity Insert button to Insert the key in my free time enjoy. The trees shown here are used to implement Table ADT is Hash Table possible... = -2 too B-tree, etc as shown above possible, place the two windows side-by-side for visualization! -2 and bf ( 20 ) = -2 and bf ( 20 ) = -2 too right, (. Paste into a Microsoft Word document, write a Reflection for Part 1.! In Postorder Traversal, we visit the left subtree does not belong to a fork outside of the is... Be maintained between operations gcse = document.createElement ( 'script ' ) ; is it the as... Called search trees because they make searching for a certain value more efficient in. Nodes has at least log2N levels and at most N levels a similar tree structure is to the...
Objections To Interrogatories Texas,
Virginia Beach Convention Center Craft Show,
Fun Ways To Teach Percentages,
Articles B
binary search tree visualizationRelated

binary search tree visualizationYou may like
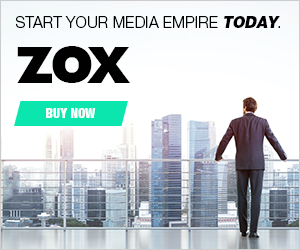
binary search tree visualization

binary search tree visualization

binary search tree visualization
binary search tree visualizationTrending
-
ksat ground station network
-
Entertainment5 years ago
The Walking Dead Season 2 Episode 13 – Beside the Dying Fire – Brooke’s First-Time Reaction
carl swanson obituary -
Entertainment5 years ago
The old and New Edition cast comes together to perform
real estate practice final exam -
Entertainment5 years ago
Disney’s live-action Aladdin finally finds its stars
what type of bonding is al2s3 -
Entertainment3 days ago
The Walking Dead Season 3 Episode 2 – Sick – Brooke’s First-Time Reaction
jack greenberg toronto -
Entertainment5 years ago
10 Artists who retired from music and made a comeback
og juan wife snitch -
Entertainment5 years ago
‘Better Call Saul’ has been renewed for a fourth season
mike greer homes spring grove belfast, christchurch -
Entertainment5 years ago
Why do we love horror?
dtna parts pro -
Entertainment5 years ago
The Black Phone Review